1、代码如下;
package com.example.forestry.util.geotools;
import org.geotools.data.DataStore;
import org.geotools.data.DataStoreFinder;
import org.geotools.data.FeatureWriter;
import org.geotools.data.Transaction;
import org.geotools.data.shapefile.ShapefileDataStore;
import org.geotools.data.simple.SimpleFeatureCollection;
import org.geotools.data.simple.SimpleFeatureIterator;
import org.geotools.data.simple.SimpleFeatureSource;
import org.geotools.jdbc.JDBCDataStore;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.simple.SimpleFeatureType;
import java.io.File;
import java.nio.charset.Charset;
import java.util.HashMap;
import java.util.Map;
public class Shp2Pgsql {
* 获取数据库连接对象
* @return
* @throws Exception
public JDBCDataStore getDataStore(){
Map<String, Object> params = new HashMap<>();
params.put("dbtype", "postgis");
params.put("host", "192.168.xx.xx");
params.put("port", 5432);
params.put("schema", "public");
params.put("database", "cim");
params.put("user", "postgres");
params.put("passwd", "postgres");
JDBCDataStore dataStore = null;
try {
DataStore ds = DataStoreFinder.getDataStore(params);
if(ds==null){
System.out.println("连接postgres失败");
}else {
dataStore = (JDBCDataStore) ds;
System.out.println("连接postgres成功");
}catch (Exception e) {
e.printStackTrace();
System.out.println("连接postres出错");
return dataStore;
* 读取shapefile文件
* @param file
* @return
public SimpleFeatureSource readShp(File file){
SimpleFeatureSource featureSource = null;
ShapefileDataStore shpDataStore = null;
try {
shpDataStore = new ShapefileDataStore(file.toURL());
Charset charset = Charset.forName("UTF8");
shpDataStore.setCharset(charset);
String tableName = shpDataStore.getTypeNames()[0];
featureSource = shpDataStore.getFeatureSource(tableName);
} catch (Exception e) {
e.printStackTrace();
} finally {
shpDataStore.dispose();
return featureSource;
* 创建表
* @param ds
* @param source
* @return
public JDBCDataStore createTable(JDBCDataStore ds , SimpleFeatureSource source){
SimpleFeatureType schema = source.getSchema();
try {
ds.createSchema(schema);
}catch (Exception e){
e.printStackTrace();
System.out.println("创建postgres数据表失败");
return ds;
* 将shapefile的数据存入数据表中
* @param ds
* @param source
public void shp2Table(JDBCDataStore ds , SimpleFeatureSource source){
SimpleFeatureIterator features = null;
String tableName = source.getSchema().getTypeName();
try {
FeatureWriter<SimpleFeatureType, SimpleFeature> writer = ds.getFeatureWriter(tableName, Transaction.AUTO_COMMIT);
SimpleFeatureCollection featureCollection = source.getFeatures();
features = featureCollection.features();
while (features.hasNext()) {
try {
writer.hasNext();
SimpleFeature next = writer.next();
SimpleFeature feature = features.next();
for (int i = 0; i < feature.getAttributeCount(); i++) {
next.setAttribute(i, feature.getAttribute(i));
writer.write();
} catch (Exception e) {
e.printStackTrace();
System.out.println("添加数据的方法错误:" + e.toString());
continue;
writer.close();
System.out.println("导入成功");
}catch (Exception e){
e.printStackTrace();
System.out.println("创建写入条件失败:"+e.toString());
}finally {
ds.dispose();
features.close();
public static void main(String[] args) {
Shp2Pgsql shp2Pgsql = new Shp2Pgsql();
File file = new File("D:\\data\\shapefile\\tree\\tree.shp");
Long startTime = System.currentTimeMillis();
JDBCDataStore dataStore = shp2Pgsql.getDataStore();
SimpleFeatureSource simpleFeatureSource = shp2Pgsql.readShp(file);
JDBCDataStore ds = shp2Pgsql.createTable(dataStore, simpleFeatureSource);
shp2Pgsql.shp2Table(ds , simpleFeatureSource);
Long endTime = System.currentTimeMillis();
Long time = (endTime - startTime)/1000;
System.out.println("时间为: "+time+" 秒");
2、插入速度;我电脑i5-10th的cpu,8G内存,跑49万条数据用了255秒;
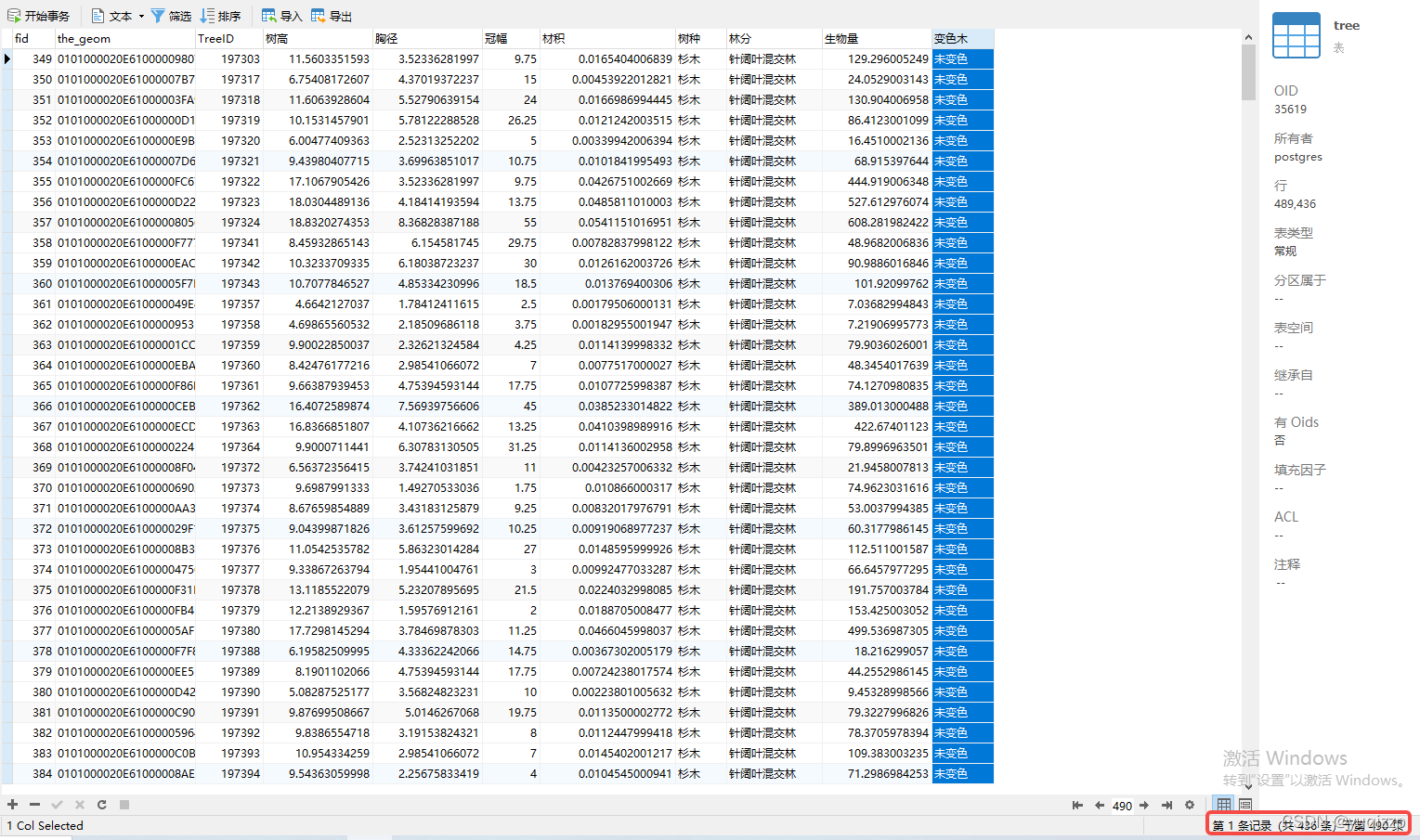
文章版权由作者李晓晖和博客园共有,若转载请于明显处标明出处:http://www.cnblogs.com/naaoveGIS/
项目中需要在浏览器端直接上传SHP后服务端进行数据的自动入PG库以及发布至geoserver。本方法是以geotools为开发工具实现入库,以geoserver manager来实现服务的自动发布。这里着重描述geotools编写SHP入库的方法。
2.Geot...
1. geotools简介
geotools是java语言封装的空间数据框架。类似于spring之与java web项目,geotools提供丰富的GIS组件,可用于快速二次搭建GIS平台。详细可登录geotools官网了解其功能信息(https://geotools.org/)。
2. geotools postgis源码解析
postgis是postgresql数据库的扩展,可以理解为postgresql的GIS化功能封装。
本博文用postgresql 11和postgis 3.1.1版本给大
GeoTools是一个开放源代码(LGPL)Java代码库,它提供了符合标准的方法来处理地理空间数据,例如实现地理信息系统(GIS)。
参照官网:https://geotools.org/
我使用的是idea
其实我创建的时候并不是按照官网上的步骤来的,因为我比较习惯从https://start.spring.io/
上面构建一个新的maven项目,填写命名下载压缩包,然后在idea中打开。
pom中增加依赖包:
<properties>
首先该程序是不改变文件的任何信息,直接读取存入到mysql数据库,每次读取一个shp,需要批量读取shp到mysql数据库需要你自己在扩展一下,导入到mysql的shp要保证不能与mysql具有相同的表明。
需要的依赖(mysql)
<dependency>
<groupId>org.geotools.jdbc</groupId>
<ar...
openssl创建证书
PostgreSQL支持使用SSL连接加密客户端/服务器通信,以提高安全性。这要求在客户端和服务器系统上都安装OpenSSL,并且在构建时启用PostgreSQL中的ssl支持(使用源码安装时的--with-openssl参数)。
首先为了使ssl工作起来,服务端首先需要配置三个参数:
ssl = on
ssl_cert_file = ...
postgresql.conf文件:
检查listen_addresses是=*(在某些postgres版本中默认为localhost)如果不是,则必须将其更改为*. 它用于监听所有接口。
pg_hba.conf文件:
添加新行:
host all all 0.0.0.0/0 md5
重新连接,正常运行
上期我介绍了geotools读取shp文件的事例 (geotools系列1-读取shp文件),本次说明读取读取postgis里表数据的代码,依旧是读取数据打印出来展示。
1、环境,maven依赖等,参见上次 geotools系列1-读取shp文件 。
2、直接上java代码
package com.jjxliu.geotools.geotools_t1;
import ...
import java.io.IOException;
import org.geotools.data.DataStore;
import org.geotools.data.DataStoreFinder;
import org.geotools.data.simple.SimpleFeatureCollection;
import org.geotools.data.simple.SimpleFeatureIterator;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.simple.SimpleFeatureType;
public class ShpFileReader {
public static void main(String[] args) throws IOException {
// 读取shp文件
File file = new File("path/to/shapefile.shp");
DataStore dataStore = DataStoreFinder.getDataStore(file);
String typeName = dataStore.getTypeNames()[0];
SimpleFeatureType schema = dataStore.getSchema(typeName);
// 获取属性表信息
System.out.println("Feature Type: " + typeName);
System.out.println("Number of attributes: " + schema.getAttributeCount());
System.out.println("Attributes: ");
for (int i = 0; i < schema.getAttributeCount(); i++) {
System.out.println(schema.getAttributeDescriptors().get(i).getName());
// 获取要素信息
SimpleFeatureCollection collection = dataStore.getFeatureSource(typeName).getFeatures();
try (SimpleFeatureIterator features = collection.features()) {
while (features.hasNext()) {
SimpleFeature feature = features.next();
System.out.println(feature.getID() + ": " + feature.getDefaultGeometryProperty().getValue());
dataStore.dispose();
需要注意的是,需要在pom.xml中添加geotools依赖:
```xml
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-shapefile</artifactId>
<version>24.0</version>
</dependency>