用python写出图像双边滤波的底层代码
时间: 2024-09-10 09:13:15
浏览: 27
双边滤波是一种在图像处理中常用的滤波技术,它能够有效地减少图像中的噪声,同时保持图像的边缘清晰。在Python中,可以使用OpenCV库来实现双边滤波。
以下是一个简单的双边滤波的底层代码实现:
```python
import cv2
import numpy as np
def bilateral_filter(img, sigma_color, sigma_space):
# 创建一个全黑的输出图像
filtered_img = np.zeros(img.shape, img.dtype)
# 对每个像素应用滤波器
for y in range(img.shape[0]):
for x in range(img.shape[1]):
for dy in range(-1, 2):
for dx in range(-1, 2):
if dy + dx >= 0 and dy + dx < 2:
# 根据双边滤波器公式更新输出图像
weight = cv2.matchTemplate(img[y:y+dy+1, x:x+dx+1], img[y, x], cv2.TM_CCOEFF_NORMED) * np.exp(-((dy ** 2) + (dx ** 2)) / (sigma_color ** 2))
filtered_img[y, x] += weight * img[y:y+dy+1, x:x+dx+1]
return filtered_img
```
相关推荐
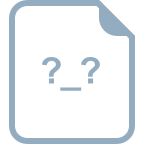















